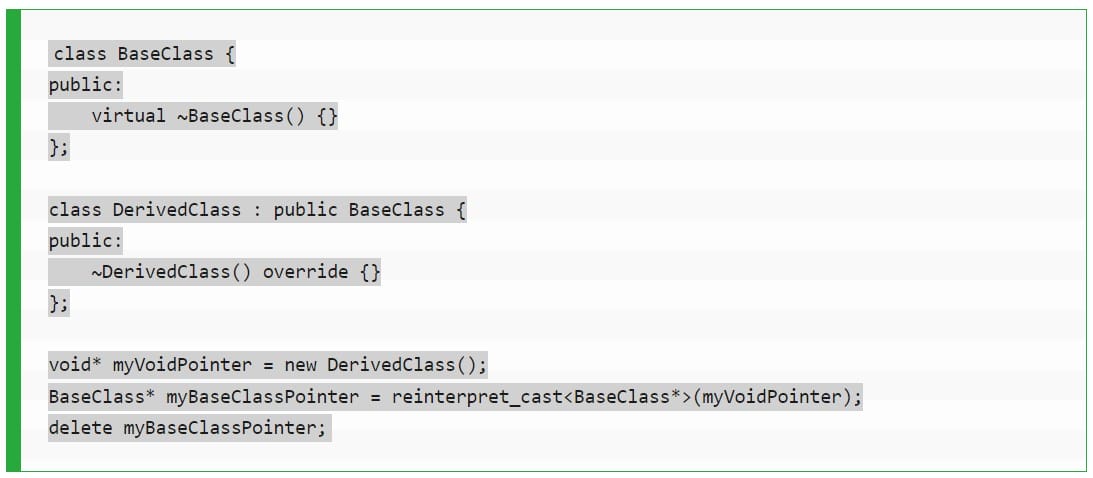
In the world of C++, pointers are a powerful tool for managing memory. They allow us to dynamically allocate and deallocate memory at runtime. However, when it comes to deleting a void pointer, things can get a bit more complicated. Void pointers, also known as generic pointers, can point to any data type but lack the type information needed for proper deletion. In this comprehensive guide, we will delve into the intricacies of deleting void pointers in C++. By the end of this article, you will have a clear understanding of how to effectively and safely delete void pointers, avoiding pitfalls such as undefined behavior.
Understanding the Void Pointer
Before we jump into the process of deleting void pointers, let’s first understand what a void pointer is. In C++, a void pointer is a special type of pointer that can hold the address of any data type. It acts as a generic pointer, allowing us to write code that can handle different types of data without explicitly specifying the type. However, this flexibility comes with a trade-off. Void pointers lack the type of information needed for proper memory management, posing a challenge when it comes to deletion.
The Challenges of Deleting a Void Pointer
When we allocate memory using a void pointer, we need to ensure that we properly deallocate that memory when we’re done using it. However, since void pointers don’t have a specific type, we can’t simply use the delete
operator to free the memory. Attempting to do so will result in a compilation error, as the compiler doesn’t know the size of the object pointed to by the void pointer.
To overcome this challenge, we need to employ some techniques to cast the void pointer to its original type before deleting it. This will provide the necessary type information to the compiler, allowing us to free the allocated memory properly.
Casting a Void Pointer to Its Original Type
To delete a void pointer, we need to cast it to its original type. This process involves converting the void pointer into a pointer of the appropriate type, which will then be used for deletion. There are two commonly used casting operators in C++: reinterpret_cast
and static_cast
.
Using reinterpret_cast
The reinterpret_cast
operator allows us to convert a pointer of one type to a pointer of any other type, regardless of the relationship between the types. However, it is important to note that reinterpret_cast
should be used with caution, as it can result in undefined behavior if used incorrectly.
Here’s an example of how we can use reinterpret_cast
to cast a void pointer to its original type before deletion:
void* myVoidPointer = new int(42);
int* myIntPointer = reinterpret_cast<int*>(myVoidPointer);
delete myIntPointer;
In this example, we first allocate memory using a void pointer and assign it the address of an integer. Then, we cast the void pointer to an integer pointer using reinterpret_cast
. Finally, we delete the integer pointer to free the allocated memory.
Using static_cast
Another option for casting a void pointer is to use the static_cast
operator. Unlike reinterpret_cast
, static_cast
performs a more restricted type conversion, ensuring type safety. It allows us to convert a pointer of one type to a pointer of a related type, such as a base class to a derived class, or vice versa.
Here’s an example of how we can use static_cast
to cast a void pointer to its original type before deletion:
void* myVoidPointer = new MyClass();
MyClass* myClassPointer = static_cast<MyClass*>(myVoidPointer);
delete myClassPointer;
In this example, we allocate memory using a void pointer and assign it the address of an object of type MyClass
. Then, we cast the void pointer to a MyClass
pointer using static_cast
. Finally, we delete the MyClass
pointer to free the allocated memory.
Handling Special Cases: Arrays and Virtual Functions
While the techniques described above work for deleting void pointers that point to single objects, there are some special cases that require additional considerations. Let’s explore how to handle arrays and objects with virtual functions.
Deleting a Void Pointer That Points to an Array
When a void pointer points to an array, we need to use the delete[]
operator instead of delete
properly deallocate the memory. The delete[]
operator ensures that all elements of the array are properly destroyed before freeing the memory.
Here’s an example of how we can delete a void pointer that points to an array:
void* myVoidPointer = new int[5];
int* myIntArray = reinterpret_cast<int*>(myVoidPointer);
delete[] myIntArray;
In this example, we allocate memory for an integer array using a void pointer and assign it to an integer pointer. Then, we use the delete[]
operator to free the memory.
Deleting a Void Pointer That Points to an Object with Virtual Functions
When a void pointer points to an object that has virtual functions, it’s important to ensure that the object’s destructor is declared as virtual. This allows for proper destruction of the object and prevents memory leaks when deleting through a base class pointer.
Here’s an example of how we can delete a void pointer that points to an object with virtual functions:
class BaseClass {
public:
virtual ~BaseClass() {}
};
class DerivedClass : public BaseClass {
public:
~DerivedClass() override {}
};
void* myVoidPointer = new DerivedClass();
BaseClass* myBaseClassPointer = reinterpret_cast<BaseClass*>(myVoidPointer);
delete myBaseClassPointer;
In this example, we allocate memory for an object of type DerivedClass
using a void pointer and assign it to a BaseClass
pointer. Then, we use the delete
operator to free the memory. Since the destructor of BaseClass
is declared as virtual, the destructor of DerivedClass
will be properly called.
Prevention is the Best Medicine
While it’s important to know how to delete void pointers, it’s even better to avoid creating them in the first place. Whenever possible, consider using specific types instead of void pointers. This allows for better type safety and avoids the complexities associated with deleting void pointers.
By using specific types, you can ensure that the appropriate delete operator is called automatically, without the need for explicit casting. This not only simplifies your code but also reduces the chances of introducing bugs related to memory management.
Deleting void pointers in C++ requires a careful understanding of type casting and memory management. By properly casting a void pointer to its original type before deletion, we can ensure that the allocated memory is freed correctly. Whether using reinterpret_cast
or static_cast
, it’s important to exercise caution and ensure that the cast is valid to avoid undefined behavior.
Remember, prevention is the best medicine. Whenever possible, avoid using void pointers and opt for specific types to simplify your code and reduce the chances of memory management issues. By following these guidelines, you can confidently handle void pointers in C++ and effectively manage your program’s memory.