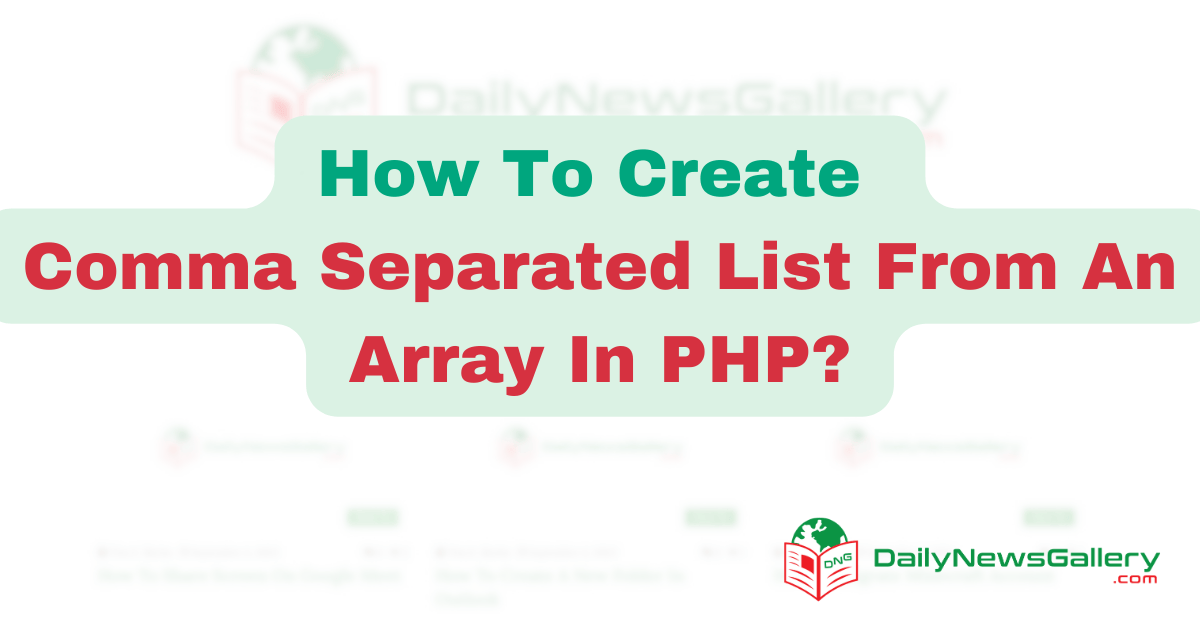
Are you an aspiring PHP developer looking to upgrade your abilities? Do you often find yourself battling to make a comma-separated list from an array in PHP? Don’t stress, this article gives you a step-by-step guide on the most proficient method to take care of this regular test. Whether you’re a beginner or have some involvement in PHP programming, realizing how to make a comma-separated list from an array is an essential aptitude that can significantly improve the effectiveness and intelligibility of your code. So, let us start and comprehend the complexities of this seemingly complex undertaking.
In PHP, arrays are a basic information structure that permits you to store various qualities inside a solitary variable. Be that as it may, with regard to speaking to these values as a comma-separated list, things can turn out to be somewhat dubious. Fortunately, PHP gives us different capacities and strategies that streamline this cycle. By following the strategies depicted in this article, you’ll have the option to effectively change over an array into a pleasantly organized comma-separated list, making your code more succinct and simpler to comprehend. So prepare to take your PHP aptitudes to the following level and open the force of making comma-separated records from arrays!
Create Comma Separated List From An Array In PHP
In this article, we will explore how to create a comma-separated list from an array in PHP. Comma-separated lists are commonly used when working with data that needs to be displayed or exported in a formatted manner. By following the step-by-step instructions provided, you will be able to efficiently convert an array into a comma-separated list using PHP.
Creating a comma-separated list from an array in PHP is a straightforward process, but there are several nuances and options you may wish to consider. Below are different scenarios and ways to handle them.
Basic Use Case with implode()
The implode()
function is the most straightforward way to create a comma-separated list from an array.
<?php
$array = array("apple", "banana", "cherry");
$commaSeparated = implode(",", $array);
echo $commaSeparated; // Output: apple,banana,cherry
?>
Adding Spaces
You can include spaces after the commas to make the list more human-readable.
<?php
$array = array("apple", "banana", "cherry");
$commaSeparated = implode(", ", $array);
echo $commaSeparated; // Output: apple, banana, cherry
?>
Nested Arrays
If your array contains other arrays (it’s multidimensional), implode()
won’t work directly. You’ll need to loop through the array and handle the nested arrays manually.
<?php
$array = array("apple", array("banana", "berry"), "cherry");
$commaSeparated = "";
foreach ($array as $item) {
if (is_array($item)) {
$commaSeparated .= implode(",", $item) . ",";
} else {
$commaSeparated .= $item . ",";
}
}
// Remove the trailing comma
$commaSeparated = rtrim($commaSeparated, ",");
echo $commaSeparated; // Output: apple,banana,berry,cherry
?>
Associative Arrays
For associative arrays, you may want to include both keys and values in the comma-separated list.
<?php
$array = array("fruit1" => "apple", "fruit2" => "banana", "fruit3" => "cherry");
$commaSeparated = "";
foreach ($array as $key => $value) {
$commaSeparated .= "$key=$value,";
}
// Remove the trailing comma
$commaSeparated = rtrim($commaSeparated, ",");
echo $commaSeparated; // Output: fruit1=apple,fruit2=banana,fruit3=cherry
?>
Null and Empty Values
By default, implode()
ignores null
and empty values. If you want to include them, you’ll need to loop through the array and append commas manually.
<?php
$array = array("apple", null, "", "cherry");
$commaSeparated = "";
foreach ($array as $item) {
$commaSeparated .= "$item,";
}
// Remove the trailing comma
$commaSeparated = rtrim($commaSeparated, ",");
echo $commaSeparated; // Output: apple,,
?>
Using Array Functions
You can also use array functions like array_map()
or array_filter()
to modify or filter the elements before imploding them.
<?php
$array = array(" apple ", "banana ", " cherry");
$trimmedArray = array_map('trim', $array);
$commaSeparated = implode(",", $trimmedArray);
echo $commaSeparated; // Output: apple,banana,cherry
?>
CSV Generation
If you’re generating a comma-separated list for use in a CSV file, consider using PHP’s built-in fputcsv()
function, as it handles edge cases (like values containing commas or newlines) automatically.
<?php
$array = array("apple", "banana, berry", "cherry\npie");
$fp = fopen('file.csv', 'w');
fputcsv($fp, $array);
fclose($fp);
?>
Step 1: Define the Array
The first step is to define the array that you want to convert into a comma-separated list. You can either manually create the array or retrieve it from a database or other data source. Here’s an example of how to manually create an array:
$data = array('apple', 'banana', 'orange', 'grape');
Make sure the array contains the values you want to include in the comma-separated list.
Step 2: Use the implode() Function
Once you have the array defined, you can use the implode() function in PHP to convert it into a comma-separated list. The implode() function takes two parameters: the separator (in this case, a comma) and the array you want to implode.
$commaSeparatedList = implode(',', $data);
After executing this line of code, the variable $commaSeparatedList will contain the comma-separated list generated from the array.
Step 3: Display or Use the Comma-Separated List
Now that you have the comma-separated list, you can choose to display it on a webpage, save it to a file, or use it in any other way that suits your needs. Here’s an example of how to display the list on a webpage:
echo $commaSeparatedList;
This will output the comma-separated list to the browser for the user to see.
Step 4: Further Customizations
Depending on your requirements, you may need to further customize the comma-separated list. For example, you might want to add additional formatting or manipulate the values before or after the implode() function is called. PHP provides various array functions that can help you achieve these customizations.
For instance, you can use the array_map() function to apply a callback function to each element of the array before imploding. This allows you to modify the values in the array before they are joined by the implode() function.
Alternatively, you can use the array_filter() function to remove any empty or null values from the array before imploding. This ensures that the resulting comma-separated list does not contain any unwanted or unnecessary elements.
Step 5: Error Handling
It’s important to handle any potential errors that may occur during the process of creating a comma-separated list from an array. For example, if the input array is empty, the implode() function will return an empty string. To avoid unexpected behavior, you can check if the array is empty before calling the implode() function and handle the situation accordingly.
Additionally, if the array contains values that need to be escaped, such as strings with commas or special characters, you should properly sanitize or escape those values to ensure the integrity and security of the resulting comma-separated list.
Conclusion
In this article, we have learned how to create a comma-separated list from an array in PHP. By following the step-by-step instructions provided, you should now be able to efficiently convert an array into a comma-separated list using PHP’s implode() function. Remember to handle any potential errors and customize the list according to your specific requirements. Happy coding!
Frequently Asked Questions
In this section, we will address some common questions related to creating a comma-separated list from an array in PHP.
Question 1: How do I create a comma-separated list from an array in PHP?
Creating a comma-separated list from an array in PHP is straightforward. You can use the implode()
function, which concatenates array elements with a specified delimiter:
$array = ["apple", "banana", "orange"];
$commaSeparatedList = implode(", ", $array);
The resulting $commaSeparatedList
will be a string containing “apple, banana, orange”.
Question 2: Can I customize the delimiter used in the comma-separated list?
Yes, you can customize the delimiter used in the comma-separated list by providing a different string as the second argument to the implode()
function. For example:
$array = ["apple", "banana", "orange"];
$customDelimiterList = implode(" | ", $array);
The resulting $customDelimiterList
will be a string containing “apple | banana | orange”.
Question 3: What if my array contains numeric values?
If your array contains numeric values, theimplode()
function will convert them to strings automatically. For example:$array = [1, 2, 3];
$commaSeparatedList = implode(", ", $array);
The resulting$commaSeparatedList
will be a string containing "1, 2, 3".
Question 4: Can I create a comma-separated list without any delimiter?
Yes, you can create a comma-separated list without any delimiter by passing an empty string as the second argument to the implode()
function:
$array = ["apple", "banana", "orange"];
$commaSeparatedList = implode("", $array);
The resulting$commaSeparatedList
will be a string containing "applebananaorange".
Question 5: How can I handle arrays with nested elements?
If your array contains nested elements, you can use a combination of array functions like array_map()
and implode()
to flatten the array before creating a comma-separated list. Here’s an example:
$array = [["apple", "banana"], ["orange"]];
$flattenArray = array_map("implode", $array);
$commaSeparatedList = implode(", ", $flattenArray);
The resulting$commaSeparatedList
will be a string containing "apple, banana, orange".
In conclusion, mastering the art of creating a comma-separated list from an array in PHP is an essential skill for any developer. As we have seen throughout this exploration, understanding the various techniques available will not only save time and effort but also enhance the overall efficiency and readability of your code. By utilizing the implode() function or employing a loop with conditional statements, you can easily transform an array into a well-formatted comma-separated list.